Switch case statements are a
substitute for long if statements that compare a variable to several integral
values. A switch statement allows a variable to be tested for
equality against a list of values. Each value is called a case, and the
variable being switched on is checked for each switch case.
Syntax:
switch(expression){
case value1
: //
code to be executed if expression = value1;
statement
1;
……………
………………
Break;//optional
case value2
: // code to be executed if expression = value2;
statement
1;
……………
………………
Break;//optional
case value3
: //// code to be executed if expression = value3;
statement
1;
……………
………………
Break;//optional
case value4
: // code to be executed if expression =
value4;
statement
1;
……………
………………
Break;//optional
case value5
: // code to be executed if
expression = value5;
statement
1;
……………
………………
Break;//optional
case valueN
: //// code to be executed if expression = valueN;
statement
1;
……………
………………
Break;//optional
Default
: // code to be executed if value of expression doesn't match
any cases
Statement1;
………………………
}
Important Points about Switch Case Statements:
- The expression provided in the switch should result in a constant value otherwise it would not be valid.
- Duplicate case values are not allowed.
- The default statement is optional.
- The break statement is used inside the switch to terminate a statement sequence. When a break statement is reached, the switch terminates, and the flow of control jumps to the next line following the switch statement.
- The break statement is optional. If omitted, execution will continue on into the next case. The flow of control will fall through to subsequent cases until a break is reached.
- The constant-expression for a case must be the same data type as the variable in the switch, and it must be a constant or a literal.
- When the variable being switched on is equal to a case, the statements following that case will execute until a break statement is reached.
- A switch statement can have an optional default case, which must appear at the end of the switch. The default case can be used for performing a task when none of the cases is true. No break is needed in the default case.
Example:
//program to create simple
calculator
#include<stdio.h>
int main(){
int num1,num2,result;
char
operator;
printf("Enter
operator(+,-,*,/,%):\n");
scanf("%c",&operator);
printf("Enter
two positive integers:\n");
scanf("%d
%d",&num1,&num2);
switch(operator){
case '+'
:
result=num1+num2;
break;
case '-'
:
result=num1-num2;
break;
case '*'
:
result=num1*num2;
break;
case '/'
:
result=num1/num2;
break;
case '%'
:
result=num1%num2;
break;
default :
printf("Invalid
operator!");
}
printf("Result
of %c on %d and %d is %d.\n",operator,num1,num2,result);
return 0;
}
Output:
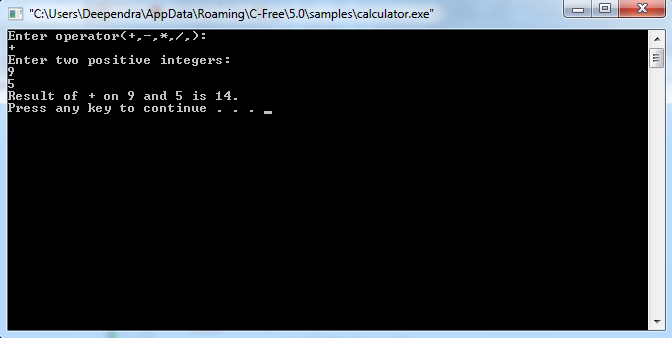
Please comment if you find anything incorrect, or you want to improve the topic discussed above.