In C, arrays can have more than one subscript. Arrays
having more than one subscript are known as multi-dimensional arrays.
Multi-dimensional
array is an array of array or more precisely collection of array.
So
Two-dimensional array is the collection of one-dimensional
array, Three-dimensional array is collection of two-dimensional array
and so on.
In this tutorial, you
will study two-dimensional arrays.
Two-dimensional array
is a collection of one-dimensional array.
They are logically represented a matrix. Any matrix problem can be converted
easily to a two-dimensional array.
Declaring 2-D array
- Like a variable, we must declare an array before using it.
- Declaring an array tells the compiler the kind of values an array will hold, number of rows and columns,
- The general syntax for declaring an array is as follows:
data_type
array_name[rows][columns];
Examples:
int
arr[3][4]; // array marks holds 10 integer
values
Initializing 2-D Array
Initializing a two-dimensional array
is similar to a one-dimensional array
with one difference. Difference is that you put the initial values for
each row between braces, {}, and then enclose all the rows between braces.
Syntax
array_name[row][columns]={
{val1,val2…},{ val1,val2…},{ val1,val2…}..
};
Example:
int arr[3][4] = {
{ 11, 12, 13, 14 }, // Values for
first row
{ 21, 22, 23, 24 }, // Values for
second row
{ 31, 32, 33, 34 } // Values for
third row
};
Each set of values that initializes
the elements in a row is between braces, and the whole lot goes between another
pair of braces. The values for a row are separated by commas, and each set of
row values is separated from the next set by a comma.
If you specify fewer initializing
values than there are elements in a row, the values will be assigned to row elements
in sequence, starting with the first. The remaining elements in a row that are
left when the initial values have all been assigned will be initialized to 0.
You can initialize the whole array to 0 by supplying just one value:
int arr[3][4] = {0};
It is important to remember that
while initializing a 2-D array it is necessary to mention the second (column)
dimension, whereas the first dimension (row) is optional. Thus the
declarations,
int arr[2][3] = { 11, 12, 13, 14,
15, 16 } ;
int arr[ ][3] = { 11, 12, 13, 14,
15, 16 } ;
are perfectly acceptable,
whereas,
int arr[2][ ] = { 11, 12, 13, 14,
15, 16 } ;
int arr[ ][ ] = { 11, 12, 13, 14,
15, 16 } ;
are invalid.
Entering data into 2-D array
Two nested loops are needed for
entering data into a 2-D array.
Syntax:
int i,j;
for(i=0;i<rows;i++){
for(j=0;j<columns:j++){
scanf(“%d”,&arr[i][j]);
}
}
Accessing elements of 2-D array
You need a nested loop to process
all the elements in a multidimensional array. The level of nesting will be the number
of array dimensions. Here’s how you could sum the elements in the previous
numbers array:
Syntax:
int i,j;
for(i=0;i<rows;i++){
for(j=0;j<columns:j++){
printf(“%d\t”,arr[i][j]);
}
printf(“\n”);
}
printf("The sum of the values
in the numbers array is %d.", sum);
Each loop iterates over one array
dimension. For each value of i, the loop controlled by j will execute
completely.
//Complete c program to read
and write data into 2-D array
#include<stdio.h>
int main()
{
int arr[3][3];
int i,j;
//Entering number into 2D array
for(i=0; i<3; i++){
for(j=0;
j<3; j++){
printf("Enter number:");
scanf("%d",&arr[i][j]);
}
}
printf("\n");
//Dislaying elements from 2D array
printf("Elements of 2D array\n");
for(i=0; i<3; i++){
for(j=0;
j<3; j++){
printf("%d\t",arr[i][j]);
}
printf("\n");
}
printf("\n");
//Dislaying address of elements of 2D array
to show that they are stored in continuous memory locations.
printf("\nFollowing addresses shows
that elements of multi dimensional array are also stored in continuous memory
locations.\n");
for(i=0; i<3; i++){
for(j=0;
j<3; j++){
printf("%d\t",&arr[i][j]);
}
}
printf("\n");
return 0;
}
Output:
Memory
representation of a 2-Dimensional Array
Like one-dimensional array, in a
two-dimensional array, the array elements are stored in one continuous memory
locations.
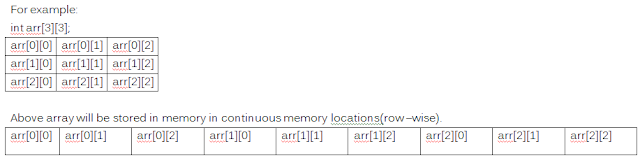
Please comment if you find anything incorrect, or you want to improve the topic discussed above.
No comments:
Post a Comment